TypeScript has gained immense popularity in the web development community for its ability to bring strong typing and enhanced tooling to JavaScript. As developers strive to build more scalable and robust applications, the demand for efficient TypeScript tooling has also surged.
In this blog post, we will explore a collection of must-have libraries and utilities that significantly improve your TypeScript development workflow. These tools cover everything from code analysis and formatting to testing and documentation, making them essential companions for any TypeScript project.
1. ESLint with TypeScript support

ESLint is a powerful linter that helps maintain code quality and enforce consistent coding standards. When working with TypeScript, it is essential to have ESLint configured with TypeScript support to catch type-related issues and other common code problems.
To set up ESLint for TypeScript we will use the typescript-eslint tooling. You need to install the following packages:
npm install eslint @typescript-eslint/parser @typescript-eslint/eslint-plugin --save-dev
Then, create an .eslintrc.js
configuration file in the root of your project:
module.exports = {
parser: '@typescript-eslint/parser',
plugins: ['@typescript-eslint'],
extends: ['eslint:recommended', 'plugin:@typescript-eslint/recommended'],
rules: {
// Add custom rules here (if necessary)
},
};
With this setup, ESLint will analyze your TypeScript code and provide valuable feedback to ensure code consistency and maintainability.
2. Prettier
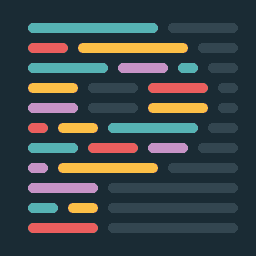
Prettier is a code formatter that automatically enforces a consistent code style across the project, ensuring that all team members adhere to the same coding standards. It works harmoniously with ESLint, and together they make an unbeatable combination for code quality.
To use Prettier with TypeScript, install the required packages:
npm install prettier eslint-config-prettier eslint-plugin-prettier --save-dev
Then, update your ESLint configuration:
module.exports = {
// ...
extends: ['eslint:recommended', 'plugin:@typescript-eslint/recommended', 'prettier'],
rules: {
// ...
'prettier/prettier': 'error',
},
};
Prettier will format your code automatically, removing the need for manual code styling efforts.
3. TypeScript Compiler
Of course, you can’t work with TypeScript without the TypeScript compiler itself. It converts TypeScript code into plain JavaScript that can run in any modern browser or Node.js environment. The TypeScript compiler also performs static type-checking, enabling you to catch potential issues early in the development process.
To install TypeScript, use:
npm install typescript --save-dev
Once installed, you can use the tsc
command to compile your TypeScript code. For more advanced configuration, create a tsconfig.json
file in your project root:
{
"compilerOptions": {
"target": "es6",
"module": "commonjs",
"outDir": "dist",
"strict": true,
// Add more options as needed
},
"include": ["src"]
}
To learn more about how to set up your Typescript configuration file. Visit here.
4. TypeScript Path Mapping
When your TypeScript project grows, managing relative import paths can become cumbersome and error-prone. TypeScript provides path mapping to simplify import statements and avoid long, nested paths.
In your tsconfig.json
file, you can define path aliases like this:
{
"compilerOptions": {
// ...
"baseUrl": "./src",
"paths": {
"@components/*": ["components/*"],
"@utils/*": ["utils/*"]
}
}
}
Now, instead of using relative paths, you can import modules using the defined aliases:
import { someFunction } from '@utils/myUtility';
import { MyComponent } from '@components/MyComponent';
This feature not only improves code readability but also simplifies refactoring.
5. Webpack
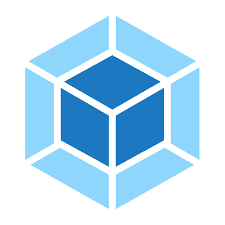
Webpack is a powerful bundler that enables you to organize and bundle your TypeScript code along with other assets like styles, images, and more. It offers various optimizations like code splitting, tree shaking, and caching to improve your application’s performance.
To set up Webpack with TypeScript, you need to install the following packages:
npm install webpack webpack-cli ts-loader --save-dev
Then, create a webpack.config.js
file in your project root:
const path = require('path');
module.exports = {
entry: './src/index.ts',
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'bundle.js',
},
resolve: {
extensions: ['.ts', '.js'],
},
module: {
rules: [
{
test: /\.ts$/,
use: 'ts-loader',
exclude: /node_modules/,
},
],
},
};
Now you can bundle your TypeScript code and other assets by running:
npx webpack
Webpack will generate a bundled JavaScript file in the dist
directory.
6. Parcel
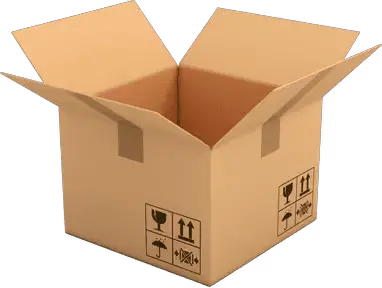
If you prefer a zero-configuration bundler, Parcel is an excellent choice. It supports TypeScript out-of-the-box and automatically handles the bundling of your assets without the need for extensive configuration.
To use Parcel with TypeScript, install the required packages:
npm install parcel@next --save-dev
Then, you can create an entry file (e.g., index.html
or index.ts
) and start the development server:
npx parcel serve src/index.ts
Parcel will bundle your TypeScript code and serve it on a development server, complete with hot module replacement for a smooth development experience.
7. Babel
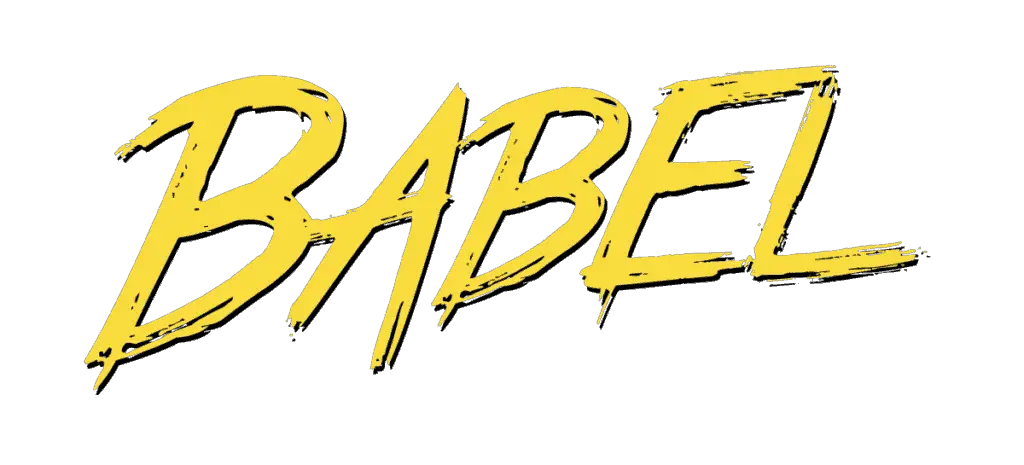
In some cases, you might need to transpile your TypeScript code to support older browsers or to use experimental features. Babel comes to the rescue by allowing you to transform TypeScript code into widely supported JavaScript versions.
To use Babel with TypeScript, install the required packages:
npm install @babel/preset-typescript @babel/core babel-loader --save-dev
Then, create a .babelrc
configuration file in your project root:
{
"presets": ["@babel/preset-typescript"]
}
To bundle your code using Babel and Webpack, update your webpack.config.js
:
module.exports = {
// ...
module: {
rules: [
{
test: /\.ts$/,
use: 'babel-loader',
exclude: /node_modules/,
},
],
},
};
This setup allows you to use the latest TypeScript features while ensuring compatibility with older browsers.
8. ts-node
When you need to execute TypeScript files directly without the need for manual compilation, ts-node is a lifesaver. It allows you to run TypeScript code directly, similar to how you would run JavaScript files with Node.js.
To use ts-node, install it globally:
npm install ts-node -g
Now you can execute your TypeScript files directly:
ts-node path/to/your-file.ts
ts-node also provides a more seamless debugging experience, making it an indispensable tool for rapid prototyping and quick experimentation.
9. Jest
Testing is an essential aspect of any software development process. Jest is a popular testing framework that works exceptionally well with TypeScript, providing an intuitive and feature-rich testing experience.
To use Jest with TypeScript, install the required packages:
npm install jest ts-jest @types/jest --save-dev
Create a jest.config.js
file in your project root to configure Jest:
module.exports = {
preset: 'ts-jest',
testEnvironment: 'node',
// Add more configuration as needed
};
With Jest and ts-jest configured, you can write tests for your TypeScript code using Jest’s powerful testing utilities.
Visit here to learn how you can use Jest for testing React applications.
10. Cypress
Cypress is a modern end-to-end testing framework that allows you to write automated tests for your web applications. It has excellent TypeScript support, which enables you to write tests with strong typings and editor autocompletion.
To set up Cypress with TypeScript, install the required packages:
npm install cypress @types/cypress --save-dev
Create a tsconfig.json
file in the cypress
directory to enable TypeScript support:
{
"compilerOptions": {
"baseUrl": "../node_modules",
"types": ["cypress"]
},
"include": ["**/*.ts"]
}
Now you can write your Cypress tests using TypeScript, taking advantage of all the features TypeScript offers.
11. Storybook
Storybook is a powerful tool for developing UI components in isolation. When working with TypeScript, Storybook provides excellent support, allowing you to document and interact with components while leveraging TypeScript typings.
To use Storybook with TypeScript, install the required packages:
npm install @storybook/react @storybook/addon-actions @storybook/addon-links @storybook/addon-essentials react-is react-docgen-typescript-loader --save-dev
Create a .storybook
directory in your project root and add a main.js
file with the following content:
module.exports = {
stories: ['../src/**/*.stories.tsx'],
addons: ['@storybook/addon-actions', '@storybook/addon-links', '@storybook/addon-essentials'],
};
In the same directory, create a webpack.config.js
file to support TypeScript files:
module.exports = async ({ config }) => {
config.module.rules.push({
test: /\.(ts|tsx)$/,
use: [
{
loader: require.resolve('ts-loader'),
},
{
loader: require.resolve('react-docgen-typescript-loader'),
},
],
});
config.resolve.extensions.push('.ts', '.tsx');
return config;
};
Now you can create stories for your components, documenting and testing them effectively with TypeScript.
12. TypeDoc
TypeDoc is an excellent tool for generating documentation from your TypeScript source code, including type information. TypeDoc converts comments into rendered HTML documentation or a JSON model. Documentation is crucial for maintaining and sharing knowledge about your codebase.
To use TypeDoc, install the required package:
npm install typedoc --save-dev
Then, run TypeDoc with the following command:
npx typedoc --out docs src
TypeDoc will generate documentation for your TypeScript project and place it in the docs
directory.
13. Husky
Husky is a popular git hook manager that allows you to run scripts before commits and pushes, ensuring that your code adheres to the project’s standards and passes all tests before it gets committed.
To use Husky, install the required package:
npm install husky --save-dev
In your package.json
, define the pre-commit and pre-push scripts:
{
"husky": {
"hooks": {
"pre-commit": "npm run lint && npm run test",
"pre-push": "npm run test"
}
}
}
Now, whenever you commit or push changes, Husky will automatically run linting and testing to prevent any potential issues from being committed or pushed to the repository.
14. Concurrently
When working on complex projects with multiple scripts running in parallel, Concurrently helps you manage these scripts from a single command. It makes running multiple npm scripts at once a breeze.
To use Concurrently, install the required package:
npm install concurrently --save-dev
In your package.json
, define your custom script that runs multiple scripts concurrently:
{
"scripts": {
"dev": "concurrently \"npm run watch:ts\" \"npm run watch:css\"",
"watch:ts": "tsc -w",
"watch:css": "parcel watch src/index.css"
}
}
With this setup, you can start both the TypeScript compiler and Parcel bundler simultaneously with a single command:
npm run dev
Conclusion
In this blog post, we’ve explored a collection of must-have libraries and utilities for TypeScript tooling. These tools significantly enhance your TypeScript development workflow, enabling you to write clean, maintainable, and efficient code. Whether you are linting, formatting, testing, bundling, or documenting, the tools mentioned here will undoubtedly make your TypeScript journey a delightful one.