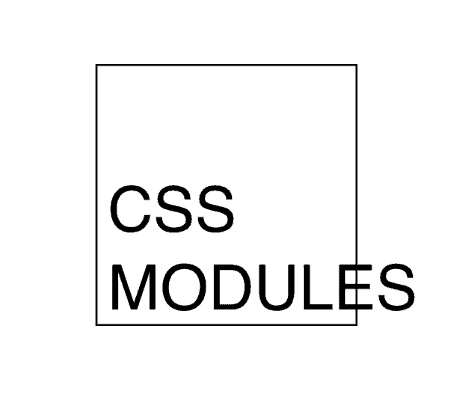
CSS (Cascading Style Sheets) is a fundamental technology for styling web pages. As web development evolves, developers are constantly seeking more efficient and maintainable ways to handle styles in their projects. One popular solution is using CSS modules, and when combined with Next.js, it becomes a powerful tool for building modern, scalable, and maintainable web applications. In this article, we will explore the world of CSS modules with Next.js and learn how it simplifies styling and provides scoped CSS for our components.
Table of Contents
- What are CSS Modules?
- Introducing Next.js
- Using CSS Modules in Next.js
- Benefits of Using CSS Modules with Next.js
- Conclusion
What are CSS Modules?
CSS Modules is a technique in web development that enables locally-scoped, modular CSS classes, preventing class name collisions and facilitating better organization and maintainability of styles.
It is a concept that allows developers to write CSS in a modular way, making it easier to manage styles in large codebases. It brings the idea of encapsulation to CSS, making styles specific to a component rather than global, thus preventing any unexpected style interference.
Traditionally, CSS classes are global, meaning that a class name declared in one component could unintentionally affect the styling of another component. This can lead to conflicts and make it challenging to maintain and refactor CSS code. With CSS Modules, each CSS file gets transformed into a local scope by default. The class names are hashed or transformed to ensure uniqueness, and they become accessible only within the component they are imported into. This way, CSS Modules promote a more organized and robust styling approach.
There are many more options for managing CSS in React.js/Next.js applications. Visit here to learn more.
Introducing Next.js
Next.js is a popular React framework that aims to simplify the process of building production-ready React applications. It provides features like server-side rendering, static site generation, code splitting, and much more out of the box, making it an excellent choice for creating complex web applications. Next.js’s built-in support for CSS Modules makes it a powerful combination for scalable and maintainable styling.
Visit here for an in-depth guide on Next.js.
Using CSS Modules in Next.js
Next.js makes it incredibly easy to use CSS Modules in your project. All you need to do is follow some conventions, and it will take care of the rest.
Creating a Component with CSS Modules
Let’s create a simple React component and demonstrate how to use CSS Modules. Inside the components
folder, create a new file named Button.js
and add the following code:
import React from 'react';
import styles from './Button.module.css';
const Button = ({ children }) => {
return <button className={styles.button}>{children}</button>;
};
export default Button;
In the above code, we import the CSS module named Button.module.css
and use the styles.button
class to style our button component. Notice that we are accessing the styles using the styles
object, which is automatically generated by Next.js when we import the CSS file with the .module.css
extension.
Styling the Button Component
Now, let’s add some styling to our button. Inside the same components
folder, create a new file named Button.module.css
and add the following code:
.button {
padding: 10px 20px;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 4px;
cursor: pointer;
}
.button:hover {
background-color: #0056b3;
}
In this CSS module, we define styles for the .button
class that we used in the Button.js
component. The styles are now scoped to this component and won’t affect other components.
Using the Button Component
Now that we have our Button
component with its styles, let’s use it in the index.js
file inside the pages
folder. Replace the existing content with the following code:
import React from 'react';
import Button from '../components/Button';
const Home = () => {
return (
<div>
<h1>Welcome to My CSS Modules App!</h1>
<Button>Click Me!</Button>
</div>
);
};
export default Home;
Save the changes, and if you switch back to your browser, you should see the “Click Me!” button with the styles applied.
Benefits of Using CSS Modules with Next.js
Using CSS Modules with Next.js offers several benefits that enhance the development process and lead to more maintainable code.
1. Encapsulation and Scope
CSS Modules provide encapsulation by design, ensuring that styles defined in one component do not interfere with other components. The hashed class names guarantee uniqueness and avoid any unintended style overrides, making it easier to reason about styles and maintain codebases.
2. Reusability and Maintainability
With CSS Modules, styles are inherently reusable, as they are tied to specific components. Developers can create a library of components, each with its unique styling, and reuse them across different parts of the application. This promotes modular and maintainable code, reducing the risk of code duplication.
3. Class Composition
Another advantage of CSS Modules is the ability to compose classes, allowing you to combine multiple styles and create new ones. This feature encourages a more flexible and efficient approach to styling, especially when building complex user interfaces.
4. Improved Performance
By generating unique class names and optimizing CSS at build time, Next.js ensures that only the required styles are loaded for each component. This reduces the overall CSS file size and improves the application’s performance by reducing the time it takes to load the page.
5. Developer-Friendly Tooling
Next.js integrates seamlessly with CSS Modules, providing a smooth development experience for developers. The autogenerated styles
object and class name hashing save developers from having to manually manage class names, leading to a more efficient and less error-prone development process.
Conclusion
CSS Modules with Next.js bring a new level of organization and maintainability to styling in modern web development. By localizing styles to specific components, CSS Modules prevent global conflicts and make it easier to manage styles in large codebases. With Next.js’s built-in support for CSS Modules, using them in your projects is seamless, providing benefits like encapsulation, reusability, and improved performance.